Regex for UK Post Code Validation implemented via JavaScript
- Jon Russell
- Jun 10, 2024
- 2 min read
Morning all
How are you?
This is one of these posts where I need to write it down more for myself than anyone else, or I’ll forget it and have to ask someone every time how to do it.
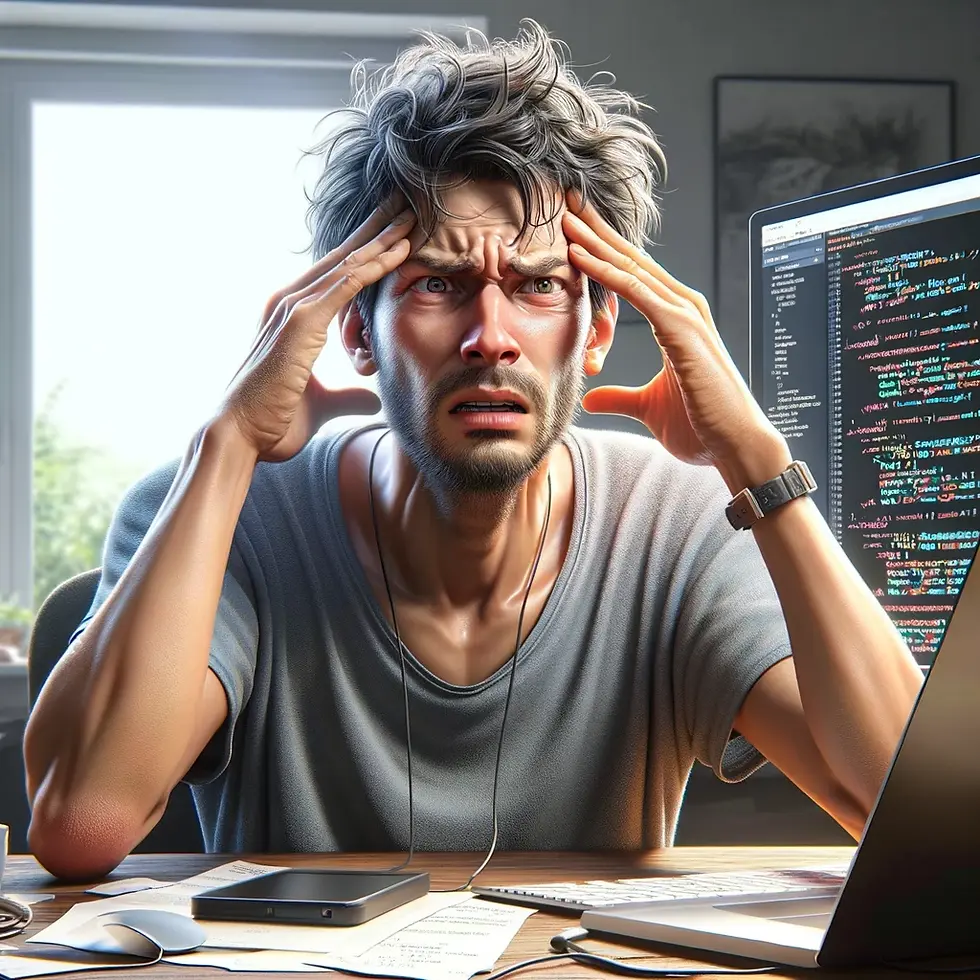
The use case is that I need to do some form validation on a UK postcode field and I need to do it in real time. To do this we need to create a regex for UK Post Code Validation implemented via JavaScript.
What is regex ?
Regex, short for regular expressions, is a tool for finding specific patterns in text. Think of it as a search tool on steroids. You use it to locate things like phone numbers, email addresses, or any other specific text format in a large document or data. It’s like a supercharged "find" command that can look for patterns, not just exact matches.
Step-by-Step Guide to Implement JavaScript Validation:
Create a JavaScript Web Resource:
Go to your Power Platform environment.
Navigate to Solutions, and add a new Web Resource.
Create a new JavaScript Web Resource (e.g., validatePostalCode.js) and add the following code.
Replace the location field value with your logical name of your field.
function validateUKPostalCode(executionContext) {
var formContext = executionContext.getFormContext();
var locationField = formContext.getAttribute("location").getValue();
// UK Postal Code Regex
var postalCodePattern = /^([A-Z]{1,2}[0-9][A-Z0-9]?)\s?[0-9][A-Z]{2}$/i;
if (!postalCodePattern.test(locationField)) {
formContext.getControl("location").setNotification("Invalid UK postal code format", "postalCodeError");
} else {
formContext.getControl("location").clearNotification("postalCodeError");
}
}
Add the Web Resource to the Form
Open form where you want to validate the postal code.
Go to Form Properties.
Add the JavaScript Web Resource (validatePostalCode.js) to the form libraries.
Configure Event Handlers:
In the Form Properties, add an event handler for the OnChange event of the Location field.
Set the function name to validateUKPostalCode and ensure the Pass execution context as first parameter option is checked.
Save and publish your changes to the form.
Thanks so much for reading. Have a great day (future me, or whoever is reading this).
Comments